8.6 Writing the Sketch
The sketch for this project borrows from ideas we encoded in two other projects. The sensor readings come from the Tweeting Bird Feeder, and the state machine for the open or closed status was copied from the Water Level Notifier. As such, here is the complete sketch.
CurtainAutomation/CurtainAutomation.pde | |
① | #include
<AFMotor.h> |
② | #define
LIGHT_PIN 0 |
#define
LIGHT_THRESHOLD 800 |
|
#define
TEMP_PIN 5 |
|
#define
TEMP_THRESHOLD 72 |
|
#define
TEMP_VOLTAGE 5.0 |
|
#define
ONBOARD_LED 13 |
|
|
|
③ | int
curtain_state = 1; |
int
light_status = 0; |
|
double
temp_status = 0; |
|
|
|
boolean
daylight = true; |
|
boolean
warm = false; |
|
|
|
AF_Stepper motor(100, 2); |
|
|
|
④ | void
setup() { |
Serial.begin(9600); |
|
Serial.println("Setting up Curtain
Automation..."); |
|
// Set stepper motor rotation speed to 100
RPMs |
|
motor.setSpeed(100); |
|
// Initialize motor |
|
// motor.step(100, FORWARD,
SINGLE); |
|
// motor.release(); |
|
delay(1000); |
|
} |
|
|
|
⑤ | void
Curtain(boolean curtain_state) { |
digitalWrite(ONBOARD_LED, curtain_state ? HIGH : LOW); |
|
if (curtain_state) { |
|
Serial.println("Opening
curtain..."); |
|
// Try SINGLE, DOUBLE, INTERLEAVE or
MICROSTOP |
|
motor.step(800, FORWARD, SINGLE); |
|
}
else { |
|
Serial.println("Closing
curtain..."); |
|
motor.step(800, BACKWARD, SINGLE); |
|
} |
|
} |
|
|
|
⑥ | void
loop() { |
|
|
// poll photocell value |
|
light_status = analogRead(LIGHT_PIN); |
|
delay(500); |
|
|
|
// print light_status value to the serial
port |
|
Serial.print("Photocell value =
"); |
|
Serial.println(light_status); |
|
Serial.println(""); |
|
|
|
// poll temperature |
|
int temp_reading =
analogRead(TEMP_PIN); |
|
delay(500); |
|
|
|
// convert voltage to temp in Celsius and
Fahrenheit |
|
float voltage = temp_reading *
TEMP_VOLTAGE / 1024.0; |
|
float temp_Celsius = (voltage -
0.5) * 100 ; |
|
float temp_Fahrenheit =
(temp_Celsius * 9 / 5) + 32; |
|
// print temp_status value to the serial
port |
|
Serial.print("Temperature value (Celsius) =
"); |
|
Serial.println(temp_Celsius); |
|
Serial.print("Temperature value (Fahrenheit) =
"); |
|
Serial.println(temp_Fahrenheit); |
|
Serial.println(""); |
|
|
|
if (light_status >
LIGHT_THRESHOLD) |
|
daylight = true; |
|
else |
|
daylight = false; |
|
|
|
if (temp_Fahrenheit >
TEMP_THRESHOLD) |
|
warm =
true; |
|
else |
|
warm =
false; |
|
|
|
switch (curtain_state) |
|
{ |
|
case 0: |
|
if (daylight &&
!warm) |
|
// open curtain |
|
{ |
|
curtain_state = 1; |
|
Curtain(curtain_state); |
|
} |
|
break; |
|
|
|
case 1: |
|
if (!daylight || warm) |
|
// close curtain |
|
{ |
|
curtain_state = 0; |
|
Curtain(curtain_state); |
|
} |
|
break; |
|
} |
|
} |
- ①
-
Reference the
AFMotor
library that will be used to drive the stepper motor attached to the Adafruit motor shield. - ②
-
We will define several values up front. This will make it easier to change the
LIGHT_THRESHOLD
andTEMP_THRESHOLD
values as we refine the stepper motor trigger points. - ③
-
Variables for storing curtain state—as well as the analog values of the photocell and temperature sensors two boolean variables,
daylight
andwarm
—are used in the main loop’s conditional statements to identify the status of daylight and the indoor room temperature. We also assign the number of steps per revolution (in this case, 100) and the motor shield port that the stepper motor is attached to (in this case, the second port per the wiring diagram) by creating anAF_Stepper
object calledmotor
. - ④
-
Here’s where we initialize the serial port to output the light and temperature readings to the Arduino IDE serial window, as well as initialize the speed of the motor (in this case, 100 revolutions per minute).
- ⑤
-
The Curtain function will be called when the light or temperature thresholds are exceeded. The state of the curtains (open or closed) is maintained so that the motor doesn’t keep running every second the threshold is exceeded. After all, once the curtains are opened, there’s no need to open them again. In fact, doing so might even damage the stepper motor, grooved pulley, or curtain drawstring.
If the Curtain function receives a
curtain_state
of true, the stepper motor will spin counterclockwise to open the curtains. Acurtain_state
value of false will spin clockwise to close the curtains.We will also use the Arduino’s onboard LED to indicate the status of the curtains. If the curtains are open, the LED will remain lit. Otherwise, the LED will be off. Since the motor shield will be covering the top of the Arduino, the onboard LED won’t be easily visible, but it will still serve as a good visual aid for debugging purposes.
- ⑥
-
The main loop of the sketch is where all the action happens. We poll the analog values of the photocell and temperature every second, convert the electrical value of the temperature sensor both to Celsius and—for those who have yet to convert to the metric system—Fahrenheit. If the light sensor exceeds the
LIGHT_THRESHOLD
value we assigned in the#define
section of the sketch, then it must be daytime (i.e.,daytime = true
). However, we don’t want to open the curtains if it’s already warm in the room, since the incoming sunlight would make the room even warmer. Thus, if the temperature status exceeds theTEMP_THRESHOLD
, we will keep the curtains closed until the room cools down. After checking the status of thecurtain_state
, we will pass a new state to theCurtain
routine and open or close the curtains accordingly.
Verify, download, and execute the sketch on the Arduino. Leave the Arduino tethered to your computer and open the Arduino IDE’s serial window to see the light and temperature values being captured by the sensors. Now we can verify whether exceeding the threshold values produces the desired effect of activating the stepper motor (see Figure 34, Test the Curtain Automation sketch).
Test the Curtain Automation sketch first by covering the photocell with your finger to verify that the stepper motor rotates the shaft in the counterclockwise direction. Remove your finger, and the shaft should rotate clockwise the same number of times. Blow warm air or use a blow dryer to warm up the air around the temperature sensor. When the threshold is exceeded, the stepper motor shaft should spin clockwise. This translates to open curtains being closed. Before removing the heat source, cover the photocell with your finger again. Then remove the heat source. The stepper motor shaft should remain motionless.
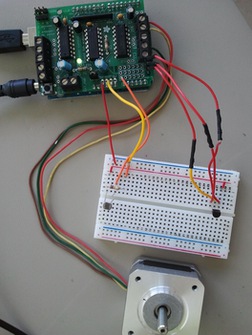
Figure 34. Test the Curtain Automation sketch
Remove your finger from the photocell. If the air surrounding the temperature sensor has cooled, the shaft should rotate counterclockwise. If it doesn’t rotate, blow air on the temperature sensor to cool it down; it should react once the temperature drops below the target threshold. Verify that your sketch reacted when the designated threshold values for light and temperature were exceeded. You may need to tweak these threshold values to ensure that the stepper motor reacts when the desired light densities and room temperature are attained. You may also need to consider omitting a light or temperature sensor range for the assigned threshold values. Otherwise, the stepper motor may act a bit jittery as the light or temperature wavers back and forth between triggered threshold values.
After you have confirmed that the sensors are properly reporting their values and the stepper motor shaft moves when the threshold values are exceeded, we can seat the sensors on a windowsill and mount the stepper motor on the wall next to the curtains. Once the system is working, you can also choose to reposition the sensors anywhere inside the room as long as the wire attaching the sensors to the Arduino board is long enough. If you do so, make sure that the wire and sensors are not in an area where they might accidentally be stepped on or where the connecting wire could be tripped over.