5.5 Going Wireless
Although more ubiquitous 802.11b/g Wi-Fi shields exist for the Arduino (such as Sparkfun’s WiFly Shield), the most prevalent means of Arduino wireless communication is via XBee radios. The initial outlay for a set of XBee devices can be a bit pricey. This is because in addition to the XBee radios, you also need an FTDI USB cable to connect one of the radios to a computer to act as a wireless serial port.
The other XBee is typically connected to an Arduino. And to make it easier to make these connections, additional kits are available to mount the XBee radios to an assembly that better exposes the connection pins while also displaying data transfers via onboard LEDs. Such visual indicators can be quite helpful when debugging or troubleshooting a paired XBee connection. Nevertheless, the fact that XBees offer a low-power and long-range (up to 150 feet) solution coupled with easy connectivity and data transfer protocol make them an ideal wireless technology for our project needs.
In order to more easily interface with the XBee radios, Adafruit has designed an adapter kit that “doesn’t suck,” but it does require you to solder a few small components onto the adapter board. Assemble the adapter following the instructions posted on Ladyada’s website.[48]
Once assembled, configuring and pairing the XBee radios isn’t too difficult, though one of the more helpful utilities used to configure them only runs on Windows.
Following the instructions for the XBee
point-to-point sample program available on Ladyada’s web
page,[49]
attach the power, ground (Gnd), receive (RX), and transmit (TX)
pins of one of the adapter-mounted XBees to the Arduino’s 5V, Gnd,
digital 2, and digital 3 pins. Plug the Arduino into your computer,
upload the test program, open up the Arduino serial window, and
make sure it is set to a rate of 9600 baud. Leave the Arduino
plugged in and attach the other paired XBee to your computer via
the FTDI USB cable. Open up a serial terminal session:
Hyperterminal in Windows, the screen
on a Mac, or various serial
communication programs such as Minicom for Linux.[50]
Once you have established a serial connection, type in a few characters in your serial application’s input screen. If your XBee radios are properly configured, you will see those typed characters appear in the Arduino serial window. If you connected the XBees to the Arduino and the FTDI cable using XBee adapters, you should also see the adapter’s green transmit and red receive LEDs blinking as the characters are wirelessly transmitted from one XBee radio to the other.
If you do not see the characters being displayed on
the receiving window, review the XBee radio wiring to the
appropriate Arduino ports. You can also swap the radios to verify
both are recognized using the FTDI USB connection. Type an
AT
in your serial application’s
terminal window and verify that it returns an OK
acknowledgment.
If the XBees still don’t seem to be communicating with one another, contact the retailer you purchased them from for further assistance.
With the XBee radios successfully paired, we will reconnect both the photocell and foil resistor to the XBee-outfitted Arduino and combine the code that will receive the sensor’s trigger condition events. For the complete wiring diagram, refer to Figure 14, Tweeting bird feeder with sensors and XBee radio attached to Arduino.

Figure 14. Tweeting bird feeder with sensors and XBee radio attached to Arduino
Consider using a breadboard, or solder the resistors along with the wires being connected to the Arduino pins. If you opt for a breadboard for testing purposes, keep in mind that it probably won’t fit in the feeder, so be prepared to solder the wiring permanently in place after the tests prove successful. And depending on the orientation of the Arduino Uno or Nano as it is positioned into the bird feeder, you may need to use straight header pins on the XBee adapter board instead of the default right-angled pins that accompany the kit. The goal is to make sure everything fits inside the feeder in a secure and serviceable way. And recall that unlike the female headers on an Arduino Uno, the Arduino Nano uses male pins instead. As such, you will need to use female jumper wires to better accommodate the connections to the Nano’s male pins.
Finishing the Sketch
We are going to poll both the foil and photocell sensors for activity, first to the Arduino IDE serial window, then—with one small replacement in our code—to the XBees. Essentially, we are simply going to combine the threshold code for the foil and photocell that we tested earlier while sending threshold alerts to the serial port of the XBee radio. Adding this logic to what we already wrote to test the perch and seed status, we can complete the sketch for the project.
TweetingBirdFeeder/TweetingBirdFeeder.pde | |
#include
<CapSense.h>; |
|
#include
<NewSoftSerial.h> |
|
|
|
#define
ON_PERCH 1500 |
|
#define
SEED 500 |
|
#define
CAP_SENSE 30 |
|
#define
ONBOARD_LED 13 |
|
#define
PHOTOCELL_SENSOR 0 |
|
// Set the XBee
serial transmit/receive digital pins |
|
NewSoftSerial XBeeSerial =
NewSoftSerial(2, 3); |
|
CapSense
foil_sensor = CapSense(10,7); // capacitive
sensor |
|
// resistor bridging digital pins 10 and
7, |
|
// wire attached to pin 7 side of
resistor |
|
int
perch_value = 0; |
|
byte
perch_state = 0; |
|
int
seed_value = 0; |
|
byte
seed_state = 0; |
|
|
|
void
setup() |
|
{ |
|
// for serial window
debugging |
|
Serial.begin(9600); |
|
|
|
// for XBee transmission |
|
XBeeSerial.begin(9600); |
|
|
|
// set pin for onboard led |
|
pinMode(ONBOARD_LED, OUTPUT); |
|
} |
|
|
|
void
SendPerchAlert(int perch_value,
int perch_state) |
|
{ |
|
digitalWrite(ONBOARD_LED, perch_state ? HIGH : LOW); |
|
if (perch_state) |
|
{ |
|
XBeeSerial.println("arrived"); |
|
Serial.print("Perch arrival event,
perch_value="); |
|
} |
|
else |
|
{ |
|
XBeeSerial.println("departed"); |
|
Serial.print("Perch departure event,
perch_value="); |
|
} |
|
Serial.println(perch_value); |
|
} |
|
|
|
void
SendSeedAlert(int seed_value,
int seed_state) |
|
{ |
|
digitalWrite(ONBOARD_LED, seed_state ? HIGH : LOW); |
|
if (seed_state) |
|
{ |
|
XBeeSerial.println("refill"); |
|
Serial.print("Refill seed,
seed_value="); |
|
} |
|
else |
|
{ |
|
XBeeSerial.println("seedOK"); |
|
Serial.print("Seed refilled,
seed_value="); |
|
} |
|
Serial.println(seed_value); |
|
} |
|
|
|
void
loop() { |
|
// wait a second each loop
iteration |
|
delay(1000); |
|
|
|
// poll foil perch value |
|
perch_value = foil_sensor.capSense(CAP_SENSE); |
|
|
|
// poll photocell value for
seeds |
|
seed_value = analogRead(PHOTOCELL_SENSOR); |
|
|
|
switch (perch_state) |
|
{ |
|
case 0: // no
bird currently on the perch |
|
if (perch_value >=
ON_PERCH) |
|
{ |
|
perch_state = 1; |
|
SendPerchAlert(perch_value, perch_state); |
|
} |
|
break; |
|
|
|
case 1: // bird
currently on the perch |
|
if (perch_value <
ON_PERCH) |
|
{ |
|
perch_state = 0; |
|
SendPerchAlert(perch_value, perch_state); |
|
} |
|
break; |
|
} |
|
|
|
switch (seed_state) |
|
{ |
|
case 0: // bird
feeder seed filled |
|
if (seed_value >=
SEED) |
|
{ |
|
seed_state = 1; |
|
SendSeedAlert(seed_value, seed_state); |
|
} |
|
break; |
|
|
|
case 1: // bird
feeder seed empty |
|
if (seed_value <
SEED) |
|
{ |
|
seed_state = 0; |
|
SendSeedAlert(seed_value, seed_state); |
|
} |
|
break; |
|
} |
|
} |
Note the references to the capacitive sensing and new software serial libraries at the beginning of the sketch. We initialize the variables that will use references from those libraries along with our threshold variables. Then we set up the connections to the serial window and XBee radio along with the Arduino onboard LED on pin 13. Once initialized, the program simply runs a loop and waits for the perch or seed thresholds to be exceeded or reset. If a change condition is detected, the running sketch will transmit these trigger messages to the Arduino IDE’s serial window as well as to the XBee radio.
With both the foil and photocells correctly reporting their values, redirect serial output from the Arduino IDE serial window to the XBee attached to the Arduino. Open up the serial application window to the FTDI-connected XBee, and if all goes well, the data you saw being displayed in the Arduino IDE serial window should now be showing up in the FTDI-connected serial application window on your computer. Isn’t wireless communication cool?
At this point, the hardware for our project is connected and tested and may look similar to the bird feeder components I assembled in Figure 15, A pet bird can help out with the testing and debugging of threshold values that trigger perch landing and departure events.
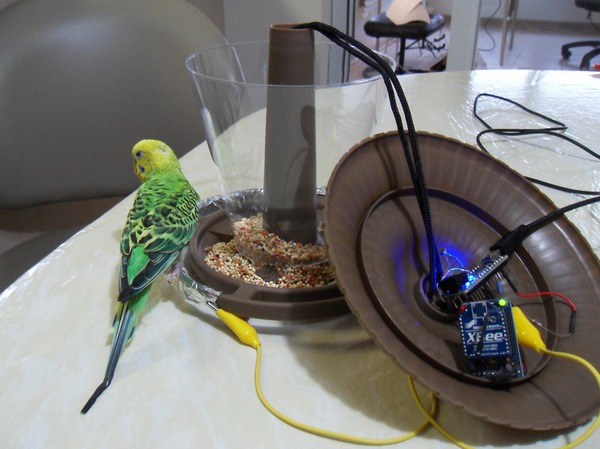
Figure 15. A pet bird can help out with the testing and debugging of threshold values that trigger perch landing and departure events.
But before we start packing the hardware into the bird feeder, we need to create one more crucial component. Using the Python language, we can write a short program that will listen for bird landings, poll for seed status, and post notable changes to Twitter. Let’s go write some code.